springboot整合tk.mybatis
程序员文章站
2022-07-15 10:10:32
...
springboot整合tk.mybatis
欢迎使用Markdown编辑器
引入pom
<dependency>
<groupId>tk.mybatis</groupId>
<artifactId>mapper</artifactId>
<version>4.1.5</version>
</dependency>
<dependency>
<groupId>tk.mybatis</groupId>
<artifactId>mapper-spring-boot-starter</artifactId>
<version>2.1.5</version>
</dependency>
目录结构
MapperScan全局扫描
package com.youmei.sh;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import tk.mybatis.spring.annotation.MapperScan;
@SpringBootApplication
//导入tk下的包
@MapperScan(“com.youmei.sh.mapper”)
public class YoumeiApplication {
public static void main(String[] args) {
SpringApplication.run(YoumeiApplication.class, args);
}
}
### 实体类
```java
package com.youmei.sh.entity;
/*轮播*/
public class Adv {
private Integer aid;
private String aimg;
private String url;
private Integer type;
private Integer port;
public Integer getAid() {
return aid;
}
public void setAid(Integer aid) {
this.aid = aid;
}
public String getAimg() {
return aimg;
}
public void setAimg(String aimg) {
this.aimg = aimg == null ? null : aimg.trim();
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public Integer getType() {
return type;
}
public void setType(Integer type) {
this.type = type;
}
public Integer getPort() {
return port;
}
public void setPort(Integer port) {
this.port = port;
}
}
mapper
package com.youmei.sh.mapper;
import com.youmei.sh.entity.Adv;
import tk.mybatis.mapper.common.Mapper;
public interface AdvMapper extends Mapper<Adv>{
}
service
package com.youmei.sh.service;
import com.youmei.sh.entity.Adv;
public interface AdvService extends BaseService<Adv> {
}
实现
package com.youmei.sh.serviceimpl;
import java.io.Serializable;
import java.util.List;
import org.apache.ibatis.session.RowBounds;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import tk.mybatis.mapper.entity.Example;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.youmei.sh.entity.Adv;
import com.youmei.sh.mapper.AdvMapper;
import com.youmei.sh.service.AdvService;
@Service
public class AdvServiceImpl implements AdvService {
@Autowired
private AdvMapper advMapper;
@Override
public int save(Adv t) {
// TODO Auto-generated method stub
return advMapper.insertSelective(t);
}
/*根据实体属性作为条件进行删除,查询条件使用等号*/
public int delete(Adv t) {
return advMapper.delete(t);
}
/*根据主键进行删除*/
public int deleteByPrimaryKey(Serializable id) {
// TODO Auto-generated method stub
return advMapper.deleteByPrimaryKey(id);
}
/*根据主键更新*/
public int update(Adv t) {
return advMapper.updateByPrimaryKeySelective(t);
}
/*根据条件更新实体*/
public int updateExample(Adv t, Example example) {
return advMapper.updateByExampleSelective(t, example);
}
/*根据Example条件进行查询*/
public List<Adv> findByExample(Example example) {
return advMapper.selectByExample(example);
}
/*条件进行查询*/
public Adv findOneExample(Example example) {
return advMapper.selectOneByExample(example);
}
/*根据id条件进行查询*/
public Adv findByPrimaryKey(Serializable id) {
return advMapper.selectOneByExample(id);
}
/*根据查询全部*/
public List<Adv> findAll() {
return advMapper.selectAll();
}
@Override
public PageInfo<Adv> findPageInfo(int pageNum, int pageSize, Example example) {
PageHelper.offsetPage(pageNum, pageSize);
List<Adv> list=advMapper.selectByExampleAndRowBounds(example, new RowBounds());
PageInfo<Adv> page=new PageInfo<Adv>(list);
return page;
}
}
控制
package com.youmei.sh.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import com.youmei.sh.entity.Adv;
import com.youmei.sh.service.AdvService;
import com.youmei.sh.util.ResultSupport;
import tk.mybatis.mapper.entity.Example;
/**
* 用户APP
* @author Administrator
*
*/
@RestController
@RequestMapping("/UserAPP")
public class AdvAppController extends CommonAppController {
@Autowired
private AdvService advService;
/**
* 查看短信验证码
* @param user
* @return
*/
@RequestMapping(value="/find",method=RequestMethod.GET)
public Map<String, Object> find(){
Map<String, Object> map = new HashMap<>();
try {
Example ex = new Example(Adv.class);
ex.createCriteria();
List<Adv> findAll = advService.findAll();
if(findAll.size()>0) {
map=new ResultSupport<Adv>(0,findAll).resultList();
}else {
map=new ResultSupport<String>(1,"系统未记录验证码").resultData();
return map;
}
} catch (Exception e) {
map = new ResultSupport<String>(2,"系统异常").resultData();
e.printStackTrace();
}
return map;
}
}
数据库
```sql
CREATE TABLE `adv` (
`aid` int(4) NOT NULL DEFAULT '0' COMMENT '图片主键',
`aimg` text COMMENT '图片url',
`type` int(1) DEFAULT '0' COMMENT '0轮播 1广告页 2启动页 3引导页',
`url` varchar(255) DEFAULT '' COMMENT '跳转链接',
`port` int(1) DEFAULT '0' COMMENT '0 用户端 1技师端',
PRIMARY KEY (`aid`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
### 效果
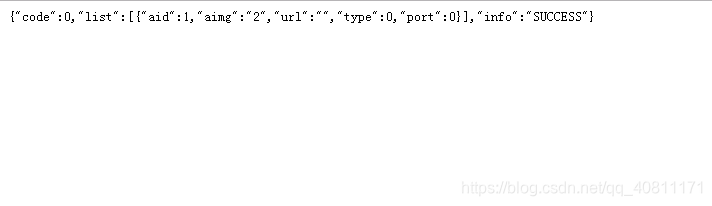
下一篇: mybatis与spring整合
推荐阅读